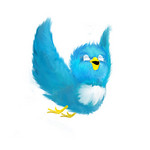

I read Hacker News, a site for startups and technologies, and occasionally post as well. A few months back, I realized that the items that I post to HN, I want to tweet as well. While I could have whipped something up with the HN RSS feed and the Twitter API (would probably be easier than Twitversation), I decided to try to use Zapier (which I’ve loved for a while). It was dead simple to set up a Zap reading from my HN RSS feed and posting to my Twitter feed. Probably about 10 minutes of time, and now I doubled my posts to Twitter.
Of course, this misses out on one of the huge benefits of Twitter–the conversational nature of the app. When my auto posts happen, I don’t have a chance to follow up, or to cc: the authors, etc.
However, the perfect is the enemy of the good, and I figured it was better to engage in Twitter haphazardly and imperfectly than not at all.