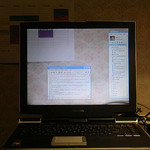

I was writing an angular app (source) that was accessing the Best Buy API, just to play around and get more familiar with Angular. All of my previous apps had been to APIs that I controlled, and could thus use CORS to set headers. Obviously, not so with the Best Buy API.
Whoops.
Luckily Angular makes accessing data via JSONP almost exactly the same as accessing data via XMLHttpRequest/CORS. Rather than use $http.get
, you use $http.jsonp
. You have the same promise returned, and can handle the results in the same way. I didn’t dive into error handling (but if Angular follows jQuery’s lead, it looks like there’s none), and obviously JSONP can only be used to read information, but the guts of injecting a script, etc, are all handled for you.