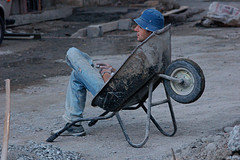

I’ve been working with a couple of REST API solutions that exist in the Java tech stack. I haven’t seen any great analysis of REST API solutions (though Matt Raible does mention some in this exhaustive slide deck about Java frameworks [pdf]), so wanted to share my on the ground experience.
First up is restSQL. This framework makes it easy to get data from a database to a JSON or XML REST API and back. If you have a servlet container available, you write two configuration files, one with a SQL query and one with db connection information, and you have a RESTful API. For prototyping and database access, it is hard to beat.
Pros:
- Quick to set up
- Only SQL knowledge is required
- No programming required
- Allows simple mapping of db table to resource, but can include one to one and one to many mappings
- Supports all four REST operations out of the box
- Supports XML as well as JSON
- Is an embeddable java library as well as a standalone framework
- Project maintainer is engaged and the project is moving forward
Cons:
- Requires a servlet engine, and you have to restart it for changes to your configuration to be picked up
- Output format has limited customization
- Only works with mysql and postgresql databases (though there is some experimental support for Oracle and MS SQL)
- Doesn’t work with views
- The security model, while fine grained, isn’t modern/OAuth (can be solved with an API gateway (like 3scale, Tyk or ApiAxle) or proxy
The next framework I have experience with is Dropwizard. This is a powerful framework that creates uberjars that you can run on any port as a standalone service. It’s not limited to providing a JSON representation of database tables–if you can create a Java object, Dropwizard can serve it up as a JSON resource.
Pros:
- Community support
- Extreme output formatting flexibility, but be prepared to write a custom deserializer if you want to handle anything other than reads of custom formatted objects
- Supports any database that hibernate supports
- Built in testing support
- Brings together ‘best of breed’ tools like Jersey, Jackson and Hibernate, so you don’t have to do the integration yourself
- Great documentation
Cons:
- Have to roll your own deployment solution (tarball, chef, puppet)
- No services startup script provided
- Shading can slow down development
- Not yet at 1.0 release
The last one I don’t have familiarity with, but a colleague used it in the past. It is Sparkjava. This is a lightweight framework that fits when you have an existing Java library with functionality you want to expose. I’m not competent to write pros/cons for this framework, but wanted to mention it.
The gorilla in the room that I haven’t had experience with (in terms of writing RESTful webs services) is Spring. I would definitely include this in any greenfield solutions review.