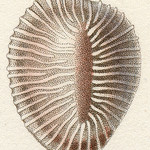

This has caused me some grief over the past few weeks, so here’s some tips on calling bash scripts from java correctly.
Use ProcessBuilder. Please.
Make sure you set the redirectErrorStream
to true, or make other provisions for handling stderr.
I’ve found that inheritIO
is useful. Not calling this caused a failure in a bash script that called other java programs.
Make sure your shell scripts have are executable, or that you call them with ‘bash scriptname’. Tarballs preserve permissions, zipfiles do not.
Read the output of the shell script, even if you do nothing with it (but you probably want to log it, if nothing else). If you don’t, buffers fill up and the process will hang:
final InputStreamReader isr = new InputStreamReader(p.getInputStream());
final BufferedReader br = new BufferedReader(isr);
String line = null;
StringBuffer sb = new StringBuffer();
while ((line = br.readLine()) != null) {
sb.append(line).append("\n");
}
Check your exit values, and wait for your shell script to finish: (If looking for speed, or more complex process flow, write it in java.)
int exitValue = p.waitFor();
if (exitValue != 0) {
// error log
}